Shape Class Referenceabstract
#include <shape.h>
Inheritance diagram for Shape:
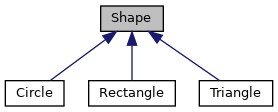
Collaboration diagram for Shape:
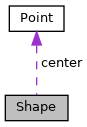
Public Member Functions | |
Shape (int x, int y) | |
Shape (Point c) | |
Point | getCenter () const |
void | setCenter (Point c) |
virtual std::string | getType () const =0 |
virtual double | getArea () const =0 |
Protected Attributes | |
Point | center |
Detailed Description
An abstract base class that represents a shape on a 2D plane. Its only member is "center" which maintains the center point of the shape. It has pure virtual methods for identifying the type of shape and calculating the shape's area that must be implemented by subclasses.
Constructor & Destructor Documentation
◆ Shape() [1/2]
Shape::Shape | ( | int | x, |
int | y | ||
) |
Overloaded constructor that takes in an (x,y) coordinate.
- Parameters
-
x The X coordinate of the shape's center point in 2D space y The Y coordinate of the shape's center point in 2D space
◆ Shape() [2/2]
Shape::Shape | ( | Point | c | ) |
Overloaded constructor that takes in a center Point.
- Parameters
-
c The center point of the shape in 2D space
Member Function Documentation
◆ getArea()
|
pure virtual |
Pure virtual function for Shape subclasses. Subclasses overriding this method should use it to calculate the area of the shape and return the area as a floating point number.
- Precondition
- Valid data must be set on the Shape object
- Postcondition
- Does not change the object
- Returns
- A floating point number representing the area of the Shape
◆ getCenter()
Point Shape::getCenter | ( | ) | const |
Accessor for the shape's center point.
- Precondition
- None
- Postcondition
- Does not change the object
- Returns
- The current center point of the shape as a Point object.
◆ getType()
|
pure virtual |
◆ setCenter()
void Shape::setCenter | ( | Point | c | ) |
Member Data Documentation
◆ center
|
protected |
The center point of the shape as a 2D coordinate
The documentation for this class was generated from the following files:
- /home/awalsh128/doxygen-themes/example_src/shape.h
- /home/awalsh128/doxygen-themes/example_src/shape.cpp