Triangle Class Reference
#include <triangle.h>
Inheritance diagram for Triangle:
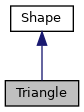
Collaboration diagram for Triangle:
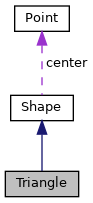
Public Member Functions | |
Triangle (int b, int h, int x, int y) | |
Triangle (int b, int h, Point c) | |
virtual std::string | getType () const |
virtual double | getArea () const |
![]() | |
Shape (int x, int y) | |
Shape (Point c) | |
Point | getCenter () const |
void | setCenter (Point c) |
Additional Inherited Members | |
![]() | |
Point | center |
Detailed Description
A class that represents a triangle on a 2D plane. Its only members are its base and height. It inherits all of its functionality from the Shape class.
Constructor & Destructor Documentation
◆ Triangle() [1/2]
Triangle::Triangle | ( | int | b, |
int | h, | ||
int | x, | ||
int | y | ||
) |
Overloaded constructor that takes in a base, a height and an (x,y) coordinate.
- Parameters
-
b The base of the triangle (must be greater than 0) h The height of the triangle (must be greater than 0) x The X coordinate of the triangle's center point in 2D space y The Y coordinate of the triangle's center point in 2D space
◆ Triangle() [2/2]
Triangle::Triangle | ( | int | b, |
int | h, | ||
Point | c | ||
) |
Overloaded constructor that takes in a base, a height, and a center Point.
- Parameters
-
b The base of the triangle (must be greater than 0) h The height of the triangle (must be greater than 0) c The center point of the triangle in 2D space
Member Function Documentation
◆ getArea()
|
virtual |
◆ getType()
|
virtual |
The documentation for this class was generated from the following files:
- /home/awalsh128/doxygen-themes/example_src/triangle.h
- /home/awalsh128/doxygen-themes/example_src/triangle.cpp